
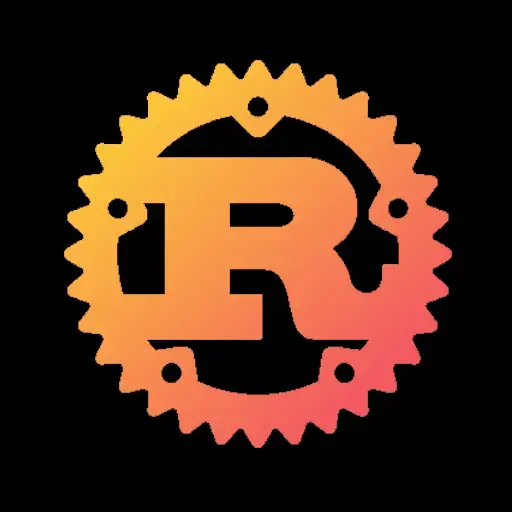
- Don’t use
"*"
dep version requirements. - Add
Cargo.lock
to version control. - Why read to string if you’re going to base64-encode and use
Vec
later anyway?
"*"
dep version requirements.Cargo.lock
to version control.Vec
later anyway?Here is an originally random list (using cargo tree --prefix=depth
) with some very loose logical grouping. Wide-scoped and well-known crates removed (some remaining are probably still known by most).
mime data-encoding percent-encoding textwrap unescape unicode-width scraper
arrayvec bimap bstr enum-iterator os_str_bytes pretty_assertions paste
clap_complete console indicatif shlex
lz4_flex mpeg2ts roxmltree speedy
aes base64 hex cbc sha1 sha2 rsa
reverse_geocoder trust-dns-resolver
signal-hook signal-hook-tokio
blocking
fs2
semver
snmalloc-rs
My quick notes which are tailored to beginners:
Option::ok_or_else()
and Result::map_err()
instead of let .. else
.let .. else
didn’t always exist. And you might find that some old timers are slightly triggered by it.Option
s as iterators (yes Option
s are iterators).?
operator and the Try
traitlet headers: HashMap = header_pairs
.iter()
.map(|line| line.split_once(":").unwrap())
.map(|(k, v)| (k.trim().to_string(), v.trim().to_string()))
.collect();
(Borken sanitization will probably butcher this code, good thing the problem will be gone in Lemmy 0.19)
Three tips here:
headers
will be returned as a struct field, the type of which is already known.collect()
itself. That may prove useful in other scenarios.Result
/Option
if the iterator items are Result
s/Option
s. So that .unwrap()
is not an ergonomic necessity 😉.into()
or .to_owned()
for &str => String
conversions.
http
crate is the compatibility layer used HTTP rust implementations. Check it out and maybe incorporate it into your
experimental/educational code.Alright, I will stop myself here.
Next Day Edit: Sorry. Forgot to use my Canadian Aboriginal syllabics again. Because apparently it’s too hard to admit HTML-sanitizing source markdown was wrong!
One thing that irks me in these articles is gauging the opinion of the “Rust community” through Reddit/HN/Lemmy😉/blogs… etc. I don’t think I’d be way off the mark when I say that these platforms mostly collectively reflect the thoughts of junior Rustaceans, or non-Rustaceans experimenting with Rust, with the latter being the loudest, especially if they are struggling with it!
And I disagree with the argument that poor standard library support is the major issue, although I myself had that thought before. It’s definitely current lack of language features that do introduce some annoyances. I do agree however that implicit coloring is not the answer (or an answer I want to ever see).
Take this simple code I was writing today. Ideally, I would have liked to write it in functional style:
async fn some_fn(&self) -> OptionᐸMyResᐸVecᐸu8ᐳᐳᐳ {
(bool_cond).then(|| async {
// ...
// res_op1().await?;
// res_op2().await?;
// ...
Ok(bytes)
})
}
But this of course doesn’t work because of the opaque type of the async block. Is that a serious hurdle? Obviously, it’s not:
async fn some_fn(&self) -> OptionᐸMyResᐸVecᐸu8ᐳᐳᐳ {
if !bool_cond {
return None;
}
let res = || async {
// ...
// res_op1()?;
// res_op2()?;
// ...
Ok(bytes)
};
Some(res().await)
}
And done. A productive Rustacean is hardly wasting time on this.
Okay, bool::then()
is not the best example. I’m just show-casing that it’s current language limitations, not stdlib ones, that are behind the odd async annoyance encountered. And the solution, I would argue, does not have to come in the form of implicit coloring.
keep in mind that it’s hard to get real numbers on LDAC because decoding is proprietary
I used to think the same. But as it turns out, a decoder exists. Maybe some people don’t want anyone to know about it to keep the myths alive ;)
EDIT: Also, as a golden rule, whenever anyone sees the words High-Res in an audio context, they should immediately realize that they are being bullshitted.
Regarding
Cargo.lock
, the recommendation always was to include it in version control for application/binary crates, but not library ones. But tendencies changed over time to include it even for libraries. If arust-toolchain
file is tracked by version control, and is pinned to a specific stable release, thenCargo.lock
should definitely be tracked too [1][2].It’s strictly more information tracked, so there is no logical reason not to include it. There was this concern about people not being aware of
--locked
not being the default behaviour ofcargo install
, giving a false sense of security/reliability/reproducibility. But “false sense” is never a good technical argument in my book.Anyway, your crate is an application/binary one. And if you were to not change the
"*"
dependency version requirement, then it is almost guaranteed that building your crate will break in the future without trackingCargo.lock
;)